Creating an Item Categories Schema with the ArcGIS API for Python (API) can be somewhat difficult to get your head around, hopefully this blog post will help with simplifying the process. In a nutshell, an Item Categories Schema enables an organization to categorize their items thematically. There can be 3 levels to a Category; the main category, a sub-category of the main category, and a sub-category of the sub-category. This depth is important to remember when it comes to creating or updating the Categories Schema with the API.
Interested in learning the ArcGIS API for Python? Get going with this course!
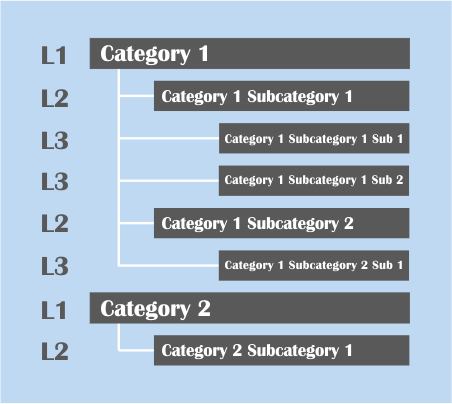
We are going to focus purely on using the API to build a Categories Schema instead of having pre-built one manually in ArcGIS Online. We start with importing the necessary module to access ArcGIS Online via the API.
from arcgis.gis import GIS
Use the GIS class to access ArcGIS Online.
agol = GIS("home")
Now we can focus on building the Categories Schema. The Categories Schema is a list containing nested dictionary objects, so let’s start with a very basic list with an empty dictionary.
categories = [{
}]
There is an overarching dictionary that contains the rest of the nested dictionaries. We’ll call this dictionary the Categories Container. The Categories Container dictionary has two key, value pairs, the first is “title” : “Categories”, and the second is “categories” : [] (pssst!! this list is what really holds the Categories Schema)
categories = [{
"title" : "Categories",
"categories" : []
}]
Within the “categories” : [] list is where we start to build the categories. Each category is a dictionary entry within this list. Each dictionary has two key, value pairs, “title” : <category name>, and “categories” : [] (this list enables us to add in sub-categories if needed).
categories = [{
"title" : "Categories",
"categories" : [{
"title" : "Category 1",
"categories" : []
}]
}]
And let’s add in a second category.
categories = [{
"title" : "Categories",
"categories" : [{
"title" : "Category 1",
"categories" : []
},{
"title" : "Category 2",
"categories" : []
}]
}]
We can now fill out the empty lists with sub-categories, we won’t go crazy, let’s just add in one for each of the two main categories so we can see what it looks like. Each sub-category is a dictionary that has two key, value pairs, “title” : <subcategory name>, and “categories” : [] (this list enables us to add in sub-categories for the sub-category if needed).
categories = [{
"title" : "Categories",
"categories" : [{
"title" : "Category 1",
"categories" : [{
"title" : "Category 1 Subcategory 1",
"categories": []
}]
},{
"title" : "Category 2",
"categories" : [{
"title" : "Category 2 Subcategory 1",
"categories": []
}]
}]
}]
And we will add in one more for each.
categories = [{
"title" : "Categories",
"categories" : [{
"title" : "Category 1",
"categories" : [{
"title" : "Category 1 Subcategory 1",
"categories": []
},{
"title" : "Category 1 Subcategory 2",
"categories": []
}]
},{
"title" : "Category 2",
"categories" : [{
"title" : "Category 2 Subcategory 1",
"categories": []
},{
"title" : "Category 2 Subcategory 2",
"categories": []
}]
}]
}]
Lastly, we can add a sub-category to a sub-category, this is as far the depth of the categories can go, therefore, there is only one key, value pair for the dictionaries at this level; “title” : <sub-subcategory name>. Let’s put in one each for a subcategory from Category 1 and 2.
categories = [{
"title" : "Categories",
"categories" : [{
"title" : "Category 1",
"categories" : [{
"title" : "Category 1 Subcategory 1",
"categories": [{
"title" : "Category 1 Subcategory 1 Sub-subcategory 1"
}]
},{
"title" : "Category 1 Subcategory 2",
"categories": []
}]
},{
"title" : "Category 2",
"categories" : [{
"title" : "Category 2 Subcategory 1",
"categories": [{
"title" : "Category 2 Subcategory 1 Sub-subcategory 1"
}]
},{
"title" : "Category 2 Subcategory 2",
"categories": []
}]
}]
}]
We’re getting the hang of it, we’ll add in a couple of more sub-subcategories.
categories = [{
"title" : "Categories",
"categories" : [{
"title" : "Category 1",
"categories" : [{
"title" : "Category 1 Subcategory 1",
"categories": [{
"title" : "Category 1 Subcategory 1 Sub-subcategory 1"
},{
"title" : "Category 1 Subcategory 1 Sub-subcategory 2"
},{
"title" : "Category 1 Subcategory 1 Sub-subcategory 3"
}]
},{
"title" : "Category 1 Subcategory 2",
"categories": []
}]
},{
"title" : "Category 2",
"categories" : [{
"title" : "Category 2 Subcategory 1",
"categories": [{
"title" : "Category 2 Subcategory 1 Sub-subcategory 1"
},{
"title" : "Category 2 Subcategory 1 Sub-subcategory 2"
}]
},{
"title" : "Category 2 Subcategory 2",
"categories": []
}]
}]
}]
Let me show you my empty Item Categories Schema in ArcGIS Online.
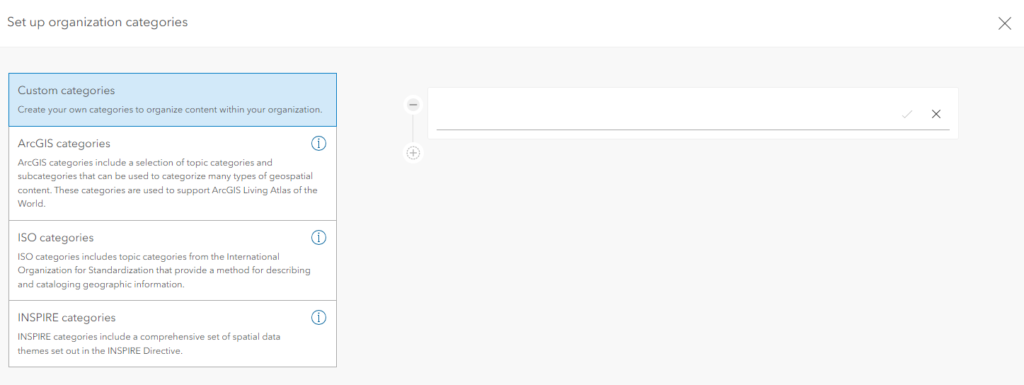
We access the categories_schema via the ContentManager and set it to be our defined categories.
agol.content.categories.schema = categories
Run the script, and we can see the update in ArcGIS Online
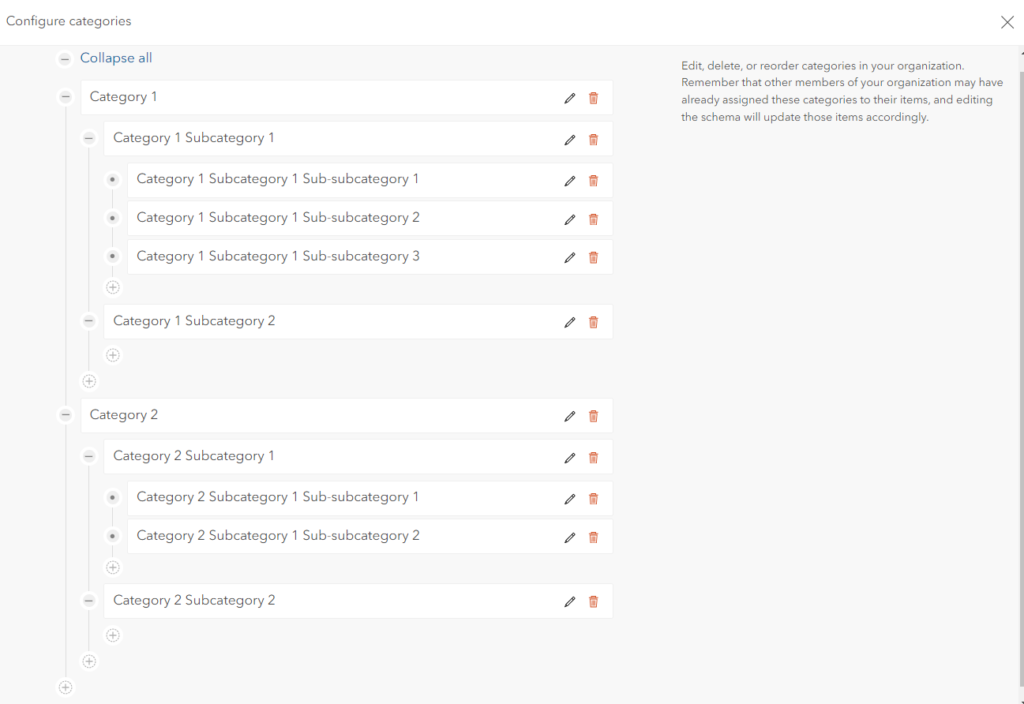
Here’s the full script.
from arcgis.gis import GIS
"""Access AGOL"""
agol = GIS("home")
""" define the schema"""
categories = [{
"title" : "Categories",
"categories" : [{
"title" : "Category 1",
"categories" : [{
"title" : "Category 1 Subcategory 1",
"categories": [{
"title" : "Category 1 Subcategory 1 Sub-subcategory 1"
},{
"title" : "Category 1 Subcategory 1 Sub-subcategory 2"
},{
"title" : "Category 1 Subcategory 1 Sub-subcategory 3"
}]
},{
"title" : "Category 1 Subcategory 2",
"categories": []
}]
},{
"title" : "Category 2",
"categories" : [{
"title" : "Category 2 Subcategory 1",
"categories": [{
"title" : "Category 2 Subcategory 1 Sub-subcategory 1"
},{
"title" : "Category 2 Subcategory 1 Sub-subcategory 2"
}]
},{
"title" : "Category 2 Subcategory 2",
"categories": []
}]
}]
}]
""" update the categories schema"""
agol.content.categories.schema = categories